Building RESTful APIs with Node.js and Express.js for Web Applications
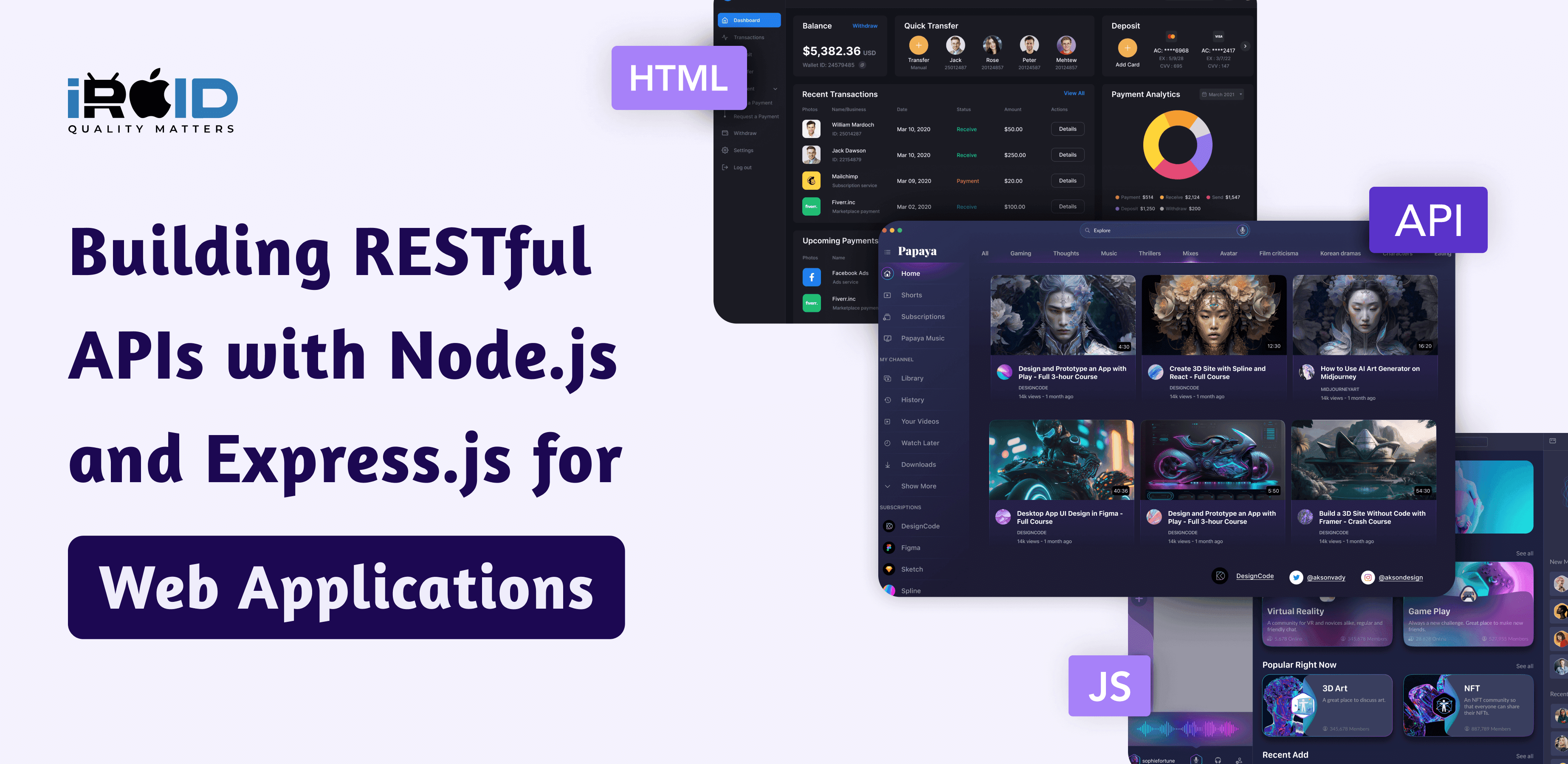
Introduction
Welcome to our comprehensive tutorial on building a REST API with Node.js and Express! In this guide, we will walk you through the process of creating a powerful and scalable API from scratch using two popular technologies: Node.js and Express.
What is a REST API?
A REST API (Representational State Transfer API) is an interface that enables the manipulation and retrieval of data over the Internet. It's designed to represent data in a format that is easily understandable by clients. REST APIs are commonly used to provide access to databases or facilitate communication between different systems and applications.
For example, consider a social media app that needs to fetch the latest posts from a server. By building a REST API, you can allow the app to send requests and receive responses containing the latest posts. This approach centralises data, making it accessible to multiple clients.
What is Node.js?
-
It's like a special JavaScript playground that lets you run code outside of web browsers, making websites and apps more powerful.
-
It's super fast and can juggle multiple tasks at once, like a skilled circus performer.
-
It's got a huge collection of tools and helpers (called modules) that other developers have shared, so you don't have to start from scratch.
What is Express?
-
It makes organising your code easier, like a good filing system for your project.
-
It handles common tasks smoothly, like setting up routes for different web pages and handling incoming requests.
-
It offers helpful tools for tasks like sending responses back to users, working with databases, and rendering HTML pages.
In this tutorial, we will leverage Node.js and the Express library to construct our REST API. Node.js is a robust JavaScript runtime for server-side applications, while Express is a popular library for building APIs and web applications with Node.js. Together, these tools offer a seamless and efficient solution for developing APIs that can handle diverse requests and workloads.
Why Node.js and Express are a winning combination for building REST APIs:
1. JavaScript Unification: Embrace the efficiency of using a single language, JavaScript, for both frontend and backend development. This streamlines your workflow, reduces context switching, and allows you to leverage your JavaScript expertise across the entire stack.
2. Performance Powerhouse: Node.js excels at handling multiple concurrent requests with ease, thanks to its event-driven architecture and non-blocking I/O model. This translates to lightning-fast API responses and exceptional scalability to handle growing user bases.
3. Express Delivery: The Express framework simplifies API development with its intuitive routing system and powerful middleware capabilities. Define routes with clarity, handle requests efficiently, and seamlessly integrate essential functionalities like authentication and logging.
4. Thriving Community: Benefit from a vast and active community of developers who continuously contribute to the Node.js and Express ecosystem. Access a wealth of libraries, resources, and support to accelerate your development process and resolve challenges effectively.
Setting up the Project:
1. Install Node.js:
- Node.js is a JavaScript runtime for server-side applications. Download and install it from [Node.js official website](https://nodejs.org/).
2. Create a new Node.js project:
- Open a terminal, navigate to your desired project directory, and run:
npm init
- Enter project information or use default values. A `package.json` file will be created.
3. Install Express:
- Install the Express library, which simplifies building APIs in Node.js:
npm install express
4. Create `app.js`:
- In your project root, create a file named `app.js`.
- Add the following code to set up Express:
javascript
5. Define a basic route:
- In `app.js`, add a simple route to handle GET requests to the root path:
javascript
6. Start the server:
- Add code to start the server in `app.js`:
javascript
- Run your API using:
node app.js
- Access it in your browser at `http://localhost:[port]`.
Defining Routes and Handling HTTP Requests:
1. Define Routes:
- Expand route handling in `app.js` by adding more endpoints for different HTTP methods (GET, POST, PUT, DELETE).
2. Handle HTTP Requests:
- Utilise `req` (request) and `res` (response) objects to handle data. Example for handling GET request to `/posts`:
javascript
Working with Data and Databases:
1. Choose a Database:
- Select a database system (e.g., MongoDB) based on your needs.
2. Connect to MongoDB (Example):
- Install Mongoose, a MongoDB driver:
npm install mongoose
- Connect to MongoDB in `app.js`:
javascript
3. Define Data Schema and Model:
- Create a schema and model for posts in `app.js`:
javascript
const Post = mongoose.model('Post', postSchema);
4. Handle Database Operations:
- Modify route to fetch posts from MongoDB:
javascript
Testing the API:
1. Install Testing Libraries:
- Install Mocha and Chai for integration testing:
npm install --save-dev mocha chai
2. Write Integration Tests:
- Create test cases for API routes using Mocha and Chai.
3. Run Tests:
- Execute tests with:
mocha
Deploying the API:
1. Choose Deployment Platform:
- Select a platform (e.g., AWS ECS, Heroku).
2. Prepare for Deployment:
- Create a Dockerfile in your project for containerization.
- Build and push Docker images to a registry (e.g., Docker Hub).
3. Deploy to AWS ECS:
- Create an AWS account and ECS cluster.
- Build a task definition and service for your API.
4. Access the Deployed API:
- Assign a public IP to your ECS tasks.
- Access your API through the assigned IP and port.
Conclusion:
Congratulations! You've successfully built a robust REST API using Node.js and Express, learned to handle HTTP requests, interact with databases like MongoDB, and even conducted integration testing. As you deploy your API, consider iRoid Solutions for expert guidance on scaling and optimising your solution. For further assistance, contact us via our website. Happy coding!
Blog Related FAQs:
A REST API (Representational State Transfer API) is a web interface that allows systems to interact by sending and receiving data using HTTP methods like GET, POST, PUT, and DELETE. It’s widely used for web and mobile applications to access and manipulate data.
Node.js offers a high-performance, non-blocking I/O model that makes it excellent for handling multiple concurrent requests efficiently. It allows developers to use JavaScript on the server side, unifying the development language for both backend and frontend.
Express is a lightweight and flexible Node.js framework that simplifies API development. It provides robust routing, middleware integration, and tools for handling HTTP requests, responses, and error management.
- Node.js: A runtime environment for running JavaScript on the server.
- Express: A framework built on top of Node.js that simplifies API and web application development with features like routing and middleware.
It depends on your project requirements:
- MongoDB: A popular NoSQL database that works seamlessly with Node.js for flexible and scalable applications.
- MySQL/PostgreSQL: Relational databases for structured data and complex queries.
Absolutely. Node.js handles concurrency effectively, and combined with tools like load balancers, Docker, or Kubernetes, you can scale your REST API to handle growing user demands.
The timeline depends on the complexity of the API. A basic API with a few endpoints can be built in a few hours, while more advanced features like authentication, database integration, and deployment may take a few days.
Recent Blog Posts
Get in Touch With Us
If you are looking for a solid partner for your projects, send us an email. We'd love to talk to you!